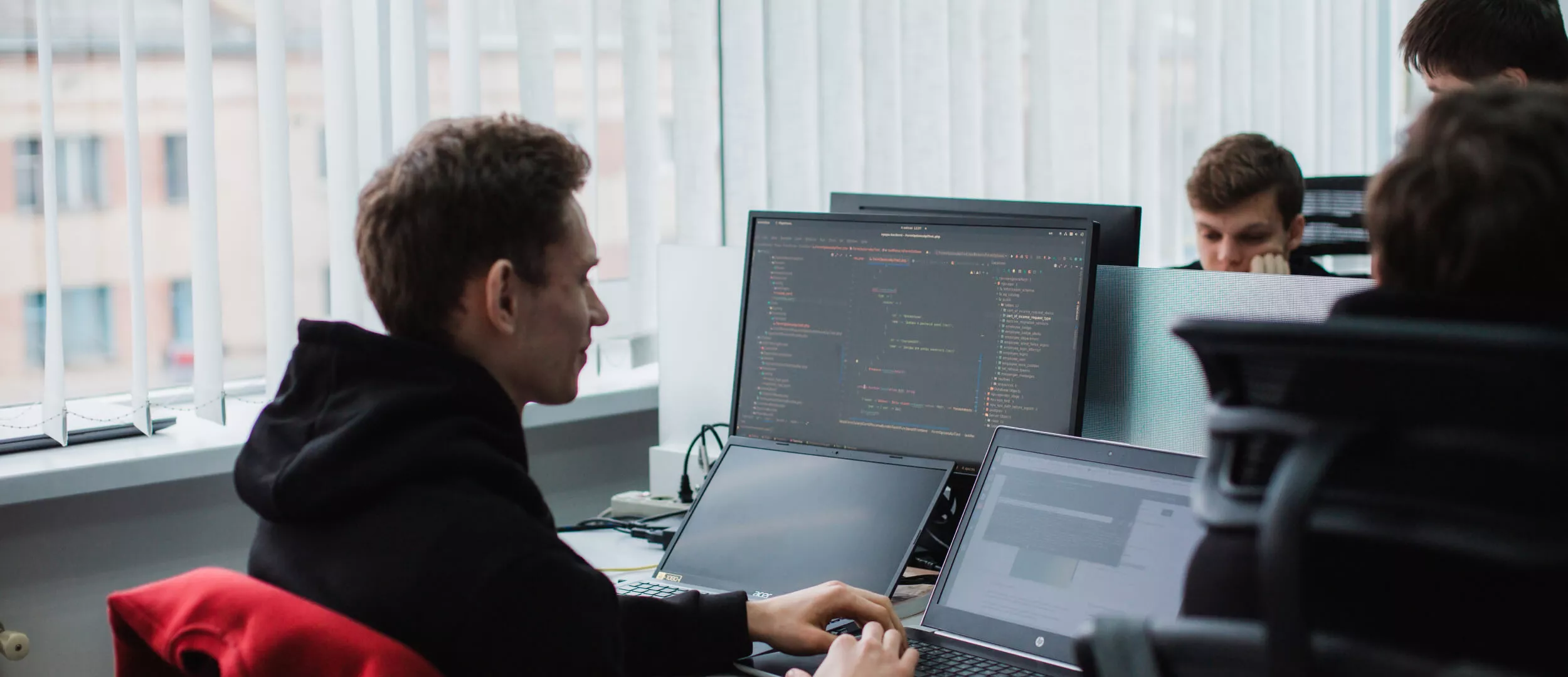
Developing elegant, robust, and performant software applications is always tricky because the field of software application development is plagued with numerous issues. Creating an application typically involves a developer running into many issues, which they must resolve. This situation is known as a Problem in a Context. The solution is always the Design Pattern.
Software design patterns are reusable solutions to recurring issues within a given context in software design. A software design pattern is not a finished design that can be directly converted to code; this is a template for solving typical problems which developers can apply in different situations.
What is a Software Design Pattern?
Design patterns are universal solutions to frequently encountered issues in software design. They resemble pre-made blueprints that you can modify to address a recurrent design issue in your code.
Software design patterns for web development offer models and techniques for developing and resolving recurrent software issues and tasks. By implementing tried-and-true patterns, software engineers can produce extensible, flexible, and high-quality code that demonstrates their mastery of the craft.
Patterns are often mistaken for algorithms because both ideas describe solutions to certain problems. A programming design pattern is a higher-level description of a solution than an algorithm that always specifies a distinct set of steps that lead to a specific outcome. The code may vary even when using the same pattern with two different programs.
Want a web app that does more?
Let's build a solution that's smart, sleek, and powerful.
Alina
Client Manager
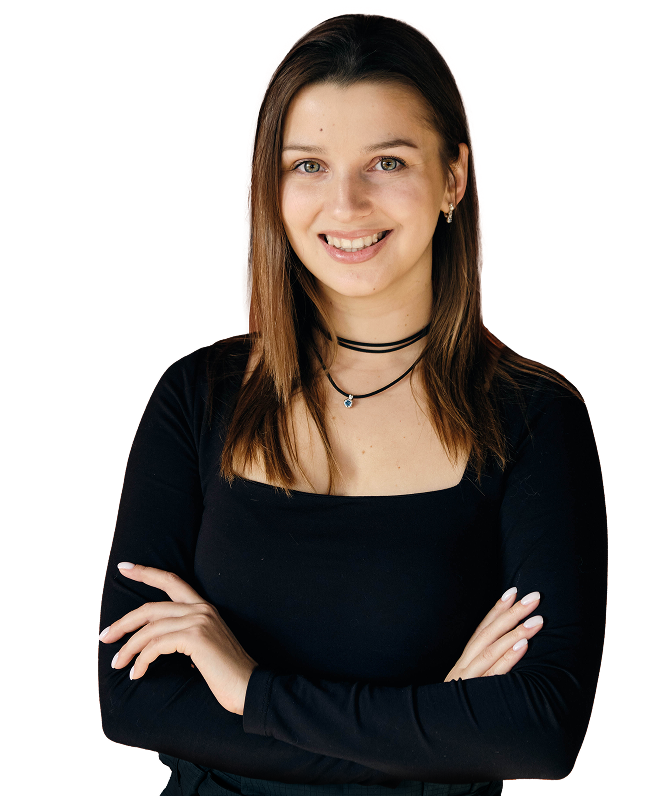
Structure of a Software Design Pattern
Most modern design patterns are described in broad strokes so that developers can use them in many contexts. The sections that make up a pattern description typically are as follows:
- The intent of the pattern describes both the issue and the solution.
- Motivation further explains the issue and how the pattern can solve it.
- The structure of classes describes each component of the pattern and how they are related.
- The code example in a popular programming language makes it easier to grasp the concept behind the pattern.
Some design pattern lists provide additional helpful information, including the applicability of the pattern, implementation stages, and connections to other patterns.
Types of Design Patterns
Software design patterns vary in complexity, level of detail, and scope of applicability to the entire system. For better understanding, this can be compared to road construction: you can make a crosswalk safer by adding traffic lights or constructing a multi-level interchange with pedestrian tunnels.
Architectural patterns are the most universal and high-level ones. Developers can use these patterns in almost any language. In contrast to other patterns, they can be used to build the architecture of the entire application.
All patterns can also be classified according to their purpose. Three primary design patterns types are:
- Creational patterns include object creation methods that improve flexibility and current code reuse.
- Structural patterns describe how to put classes into more complicated structures while maintaining efficiency and flexibility.
- Behavioral patterns ensure quick communication and the allocation of functions between objects.
- Concurrency design patterns are software design patterns used in multi-threaded programming.
Design Patterns Advantages
The software design pattern can speed up the development process. It offers tested development paradigms, saving time by preventing the need to create new patterns each time a problem arises. There are two main advantages to using design patterns.
First, they offer a way to solve software development-related problems using a tried-and-true technique. The solution makes it easier to create highly cohesive modules with little coupling. They separate any potential variations in the system requirements, which makes the entire system simpler to comprehend and maintain.
Second, design patterns improve the effectiveness of designer-to-designer communication. When discussing system design, software professionals can refer to the pattern used to solve a specific issue and instantly visualize the high-level design in their minds.
Best Software Design Patterns
Although 26 software design patterns have been discovered to date, including 23 "Gang of Four" patterns, only several are considered to be the most significant or influential. This section describes the top 7 software design patterns, their significance, and their appropriate application.
Singleton Pattern
The Singleton is a creational software design pattern that restricts the object creation of a class to a singular instance. The class has only one instance, providing a global access point to this instance.
It is one of the well-known "Gang of Four" programming patterns, which explain how to address recurrent issues in object-oriented software. The pattern is helpful when only one object is required to coordinate actions throughout a system.
An analogy from real life
The example of a government can illustrate the Singleton pattern. The legitimate government of each country is always in a single copy. The term "the Government of A" is a global access point describing a group of persons in charge.
When to apply?
- Use the Singleton design+pattern when a class should only have a single instance that is accessible to all clients; an example would be a single database object that is shared by various program components.
- Use the Singleton pattern if you need more precise control over global variables.
Pros:
- A class has a single instance
- You get a global access point to that instance
- Only first-time requests initialize the singleton object.
Cons:
- The Single Responsibility Principle is broken. The pattern simultaneously resolves two issues.
- The Singleton programming pattern may hide a poor design. For instance, when the program's components know too much about one another.
Factory Method
The Factory Method, also known as the Virtual Constructor, enables the creation of objects in a superclass while allowing subclasses to change the type of objects that are created.
New classes can be added to the factory as needed. This change might seem pointless at first glance: we moved the constructor call from one program area to another. But consider that you can now modify the class of products that the factory method creates by overriding it in a subclass.
Use the factory method
- when you're still determining what kinds and dependencies the objects your code will need to work with are;
- when you want to give users of your library or framework a way to modify its internal components;
- when you want to conserve system resources by reusing existing objects rather than rebuilding them each time.
Pros
- There is no hard link between the creator and the product.
- Single Responsibility Principle. The product creation code can be moved to one spot in the program, making it simpler to maintain.
- Open/Closed Principle. The program can add new product types without altering the client code.
Cons:
- Adding a lot of new subclasses to implement the software design pattern may make the code more complex. The scenario where you introduce the pattern into an existing hierarchy of creator classes is the best case.
Facade
Facade is a structural program design pattern that provides a streamlined interface to a complex set of classes, like a library, a framework, or any other subsystem with lots of moving parts.
It could be the perfect solution when you need to integrate your app with a complex library with dozens of features but you require a tiny portion of its functionality.
The facade programming design pattern is incredibly effective in personalization marketing strategies because it responds to a customer's location or actions to provide each user with a unique experience.
Analogy from real life
When a store customer orders over the phone, the help desk operator is a front for all department and store services. It provides the user with a simple voice interface to payment gateways, an ordering system, and delivery services.
Apply the Facade pattern
- when you need a simple yet constrained interface to a complex subsystem.
- When you want to layer a subsystem's structure.
Pros
- You can separate your code from a subsystem's complexity.
Cons
- Facades have the potential to transform into god objects connected to every app class.
Strategy
Occasionally referred to as a policy pattern, the strategy design pattern enables you to define a family of algorithms, separate each algorithm into a different class, and make the objects of each class interchangeable. For instance, a group of algorithms may be used to "sort" products on an eCommerce website according to factors like size, color, price, etc. Based on the customer's behavior, the strategy is put into practice.
The strategy design+pattern is a straightforward design pattern for personalization marketing strategies. To provide each user with a unique experience, the strategy design pattern responds to a client location, inputs, or actions.
Analogy from real life
Think about having to travel to the airport. You can ride your bicycle, order a cab, or board a bus. These are your means of transportation. You can choose one of the strategies depending on factors like your budget and time constraints.
Use the Strategy pattern
- when you need to use a variety of algorithm variants within an object and be able to switch between them while the object is being used.
- when a large number of closely related classes only vary in how they carry out a particular behavior.
- to separate an algorithm's implementation details from its business logic, which may be less critical.
Pros
- At runtime, you can change the algorithms used inside an object.
- You can separate an algorithm's implementation specifics from the code that employs it.
- You can use composition in place of inheritance.
Cons
- There is no need to add new classes and interfaces to the program associated with the pattern if you only have a few algorithms and they only change a little.
- To choose the best strategy, clients must be aware of the variations among them.
Observer
The observer is a behavioral software design pattern that enables you to specify a subscription mechanism to alert multiple objects to any events that affect the object they're watching.
The observer programming pattern establishes a one-to-many relationship between an object (the subject) and dependents (the observers). The subject is informed whenever one of the observers changes.
Any event-driven programming, such as notifying a user of a new comment on Facebook or sending an email when item ships, can benefit from using the observer design pattern.
Analogy from real life
If you subscribe to a newspaper or magazine, you can avoid going to the store to see if the upcoming issue is available. Instead, the publisher delivers fresh issues straight to your mailbox.
A list of subscribers is kept on file, and the publisher knows their magazine preferences. If subscribers no longer want the publisher to send them new magazine issues, they can remove their names from the list anytime.
Apply the Observer pattern
- when the set of objects is dynamically changing or unknown in advance, and changing the state of one object may require changing the states of other objects.
- when specific situations or for a brief period, some objects in your app need to observe others.
Pros
- Open/Closed Principle. You can add new subscriber classes without requiring changes to the publisher's code if there is a publisher interface.
- At runtime, you can build relationships between objects.
Cons
- Notification of subscribers occurs in random order.
Builder
Builder is a creational program design pattern that enables you to build complex objects piece by piece. Utilizing the same construction code, the pattern allows for producing various object types and representations.
The builder pattern calls only the steps required for each object iteration as it is constructed in a series of sequential steps.
The pattern divides the process of creating an object into several steps (buildWalls, buildDoor, etc.). The crucial point is that not all of the steps must be called. Only those processes essential for creating an object's specific configuration may be called.
Use the Builder pattern
- to eliminate a "telescoping constructor."
- when you need your code to be capable of producing various representations of a certain product.
- to assemble complex objects, such as composite trees.
Pros
- You have three options for building objects: sequentially, asynchronously, or recursively.
- You can apply the same construction code to a variety of product representations.
- Single Responsibility Principle. You can separate the product's business logic from complex construction code.
Cons
- Since the pattern necessitates the creation of numerous new classes, the overall complexity of the code rises.
Adapter
Adapter is a structural design-pattern that enables cooperation between objects with incompatible interfaces.
An adapter wraps one of the objects to conceal the intricate conversion behind the scenes. The wrapped object doesn't even know it's in an adaptive wrapper. As an illustration, you could encase a device that uses meters and kilometers in an adapter that converts all the information to imperial units like feet and miles.
Analogy from real life
You might experience a surprise when attempting to charge your laptop when visiting Europe for the first time from the US. Different countries have different standards for power plugs and sockets. The German socket won't accept your US plug because of this. A power plug adapter with both an American-style socket and a European-style plug can solve the issue.
Use the Adapter pattern
- when you use an existing class whose interface conflicts with your code.
- when creating a middle-layer class that acts as a translator between the code and a legacy class, a third-party class, or any other class with an incompatible interface.
- when you want to reuse many already-existing subclasses that don't share a common feature and are ineligible for inclusion in the superclass.
Adapter Pattern Pros
Single Responsibility Principle. You can keep the interface or data conversion code apart from the program's main business logic. Open/Closed Principle. You can add new types of adapters to the program without causing the current client code to malfunction.
Adapter Pattern Cons
Because you have to add many new classes and interfaces, the code's overall complexity rises. Sometimes it's easier to update the service class to match the rest of the code.
Architectural Patterns vs. Design Patterns
Architecture patterns set the physical locations of components, dictate how they should interact with one another, and finally, decide which tools should be used to build components.
Design patterns in software architecture should delve deeply into how to implement particular components, whereas architecture patterns focus more on the big picture. Classes, interfaces, abstract classes, and other OO features are used in component design to complete the tasks specified for each component.
The software systems are constructed according to architectural patterns. Design patterns resolve recurring issues in software design.
Architecture Patterns vs. Design Patterns:
- Architecture comes in the designing phase, and design patterns come in the building phase.
- A design pattern is the actual implementation, whereas an architectural pattern is like a blueprint.
- Architecture serves as the framework upon which all other elements are built, and design patterns organize classes to address common issues.
Final Thoughts
Having an experienced team is key to knowing when to use software design patterns—and when not to—and how to implement each pattern effectively.
Stfalcon is a leading software development company with 13 years of experience. We prioritize establishing enduring relationships with our clients and building software your company will enjoy using.
If you're looking for a dedicated software development team who will dress your idea in code, hit us up to get a free consultation from our specialists.