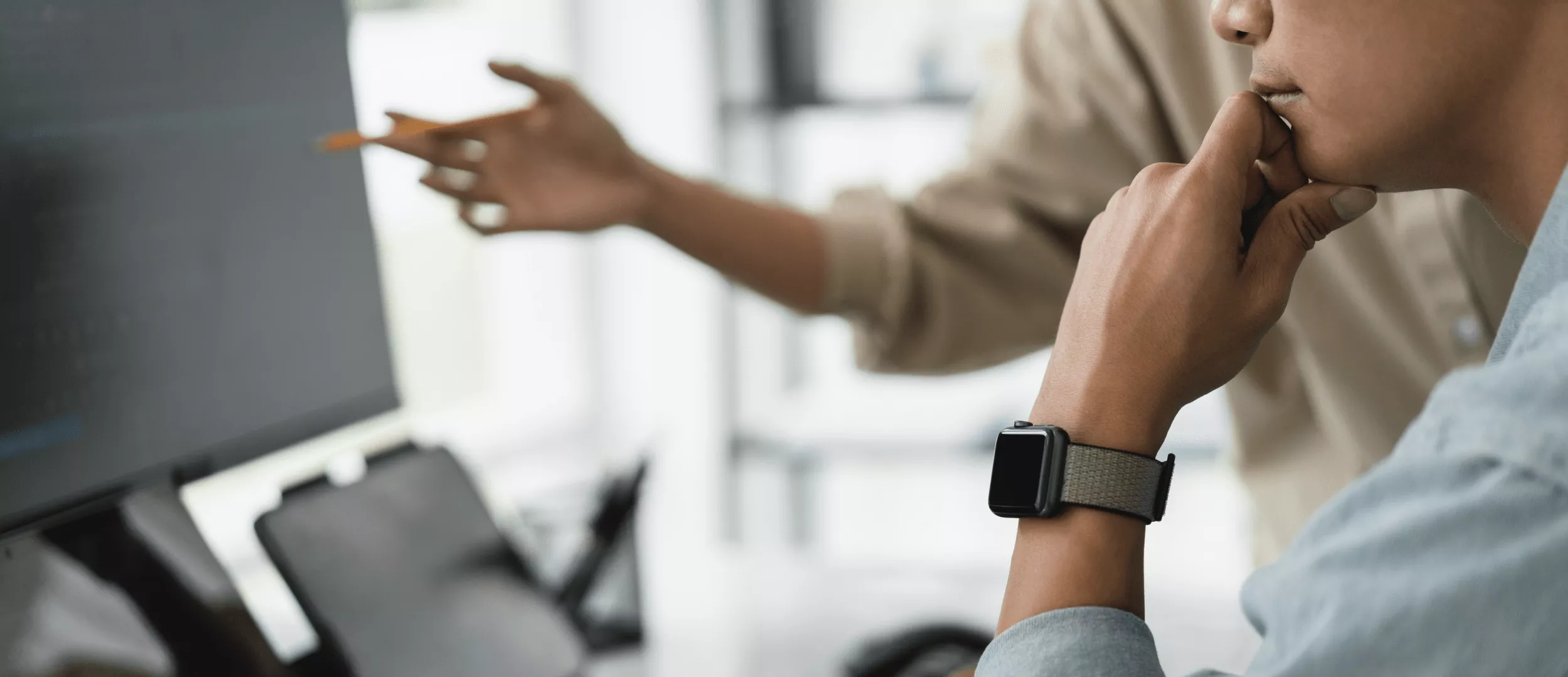
Today we would like to talk about innovations in Material Design. During Google I/O, the introduction of "Material You" was unveiled, showcasing the most captivating personalization attributes within the latest version of the Material Design system, known as Material Design 3. Material 3 represents Google's most recent iteration of its open-source design system. It empowers you to create and develop beautiful, functional products that adhere to the principles of Material Design. This update not only brings support for dynamic colors but also includes enhancements to various Material Components.
We also have an in-depth blog post on Android Material Design that you might be interested in checking out.
Material 3 encompasses refreshed theming, components, and incorporates Material You personalization elements, including dynamic color. It is meticulously crafted to harmonize seamlessly with the updated visual style and system user interface found in Android 12 and subsequent versions.
Material Theme
Themes in Material You extend the color palette beyond its previous iterations, introducing a Tertiary color and a range of interpolated colors derived from the Primary, Secondary, Tertiary, and Error color choices.
These fresh color spaces empower dynamic color expression. Visualizing dynamic colors and transitioning to our new color system is now a straightforward process with the Material Theme Builder, accessible both online and within Figma. When generated through our tools, these intermediary color values can be established with just a single input color.
Integrating Dynamic Color
To incorporate dynamic color, the DynamicColors class within the Material library leverages Activity Lifecycle Callbacks to determine when and how to apply color overlays. Utilizing the provided API calls, you have the flexibility to apply dynamic color either to specific sections or the entire app. You also have control over when and where dynamic color is applied.
To ensure unintended consequences are avoided, it's crucial that your application code references theme attributes rather than hardcoded colors (such as HEX codes or "@color/").
For an application using a Material3 theme with MDC components, the minimum code required to enable dynamic color is as follows, typically added within the onCreate method of your Application class:
java Copy code import android.app.Application; import com.google.android.material.color.DynamicColors; public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); // This is all you need. DynamicColors.applyToActivitiesIfAvailable(this); } }
Typography
Typography styles have undergone simplification. In the pre-Material 3 era, there were various headline variations, subtitle options, body styles, button text, caption text, and overline text.
In Material 3, the typography system has been refined to feature a more streamlined set of variants for each category, specifically Small, Medium, and Large.
The majority of the fresh TextAppearance styles align directly with the styles from before Material 3. In MDC-Android, the property names follow a consistent pattern: they start with "textAppearance" and then include {Display, Headline, Title, Body, Label} along with {Large, Medium, Small} as part of their names.
Component Updates
The new Carousel component offers versatile functionality, allowing you to create engaging experiences such as photo galleries and interact with lists of items more flexibly than using a RecyclerView.
Key Features:
Utilizes RecyclerView at its core, ensuring optimal performance and seamless integration with your designs.
Transitioning to a Carousel:
To implement a Carousel, you can start with an existing RecyclerView and modify your item layout files by wrapping them with a MaskableFrameLayout. These layouts will provide instructions to the Carousel on how to arrange items in relation to each other. Here's an example:
<com.google.android.material.carousel.MaskableFrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/carousel_item_container" android:layout_width="130dp" android:layout_height="match_parent" android:layout_marginStart="4dp" android:layout_marginEnd="4dp" android:foreground="?attr/selectableItemBackground" app:shapeAppearance="?attr/shapeAppearanceCornerExtraLarge"> <!-- Your item content here, e.g., ImageView --> </com.google.android.material.carousel.MaskableFrameLayout>
Instead of using LinearLayoutManager, employ a CarouselLayoutManager for your RecyclerView.
Masking and Custom Objects:
Masking in MaskableFrameLayout ranges from 0% to 100%. When masking is needed, the layout manager calculates a masking rectangle. Ensure that custom objects, such as images with custom matrices or text components, work harmoniously with Carousel's masking. You can let them respond to changes in mask size using an OnMaskChangedListener on your MaskableFrameLayout.
MultiBrowseCarouselStrategy:
This strategy optimizes the display of large, medium, and small items within the available space. It aims to determine the optimal number of large items that fit the display with minimal size adjustments. Subsequently, it incorporates medium and small items based on the available space.
At the start of the carousel, you might see two large items, a medium item, and a small item. As you scroll to the end, the arrangement may shift to one small item, followed by a medium item, and finally, two large items.
For comprehensive details on integrating the new Carousel component into your app, refer to the complete developer documentation.
Top App Bar
Top app bars have received a Material You makeover to harmonize more effectively with color schemes. Prior to Material You, top app bars relied on shadows to distinguish themselves from content. Now, newly introduced surface tones make color the primary indicator of elevation.
Navigation Bar/Bottom Navigation View
The Bottom Navigation View has received a visual update and introduced new capabilities. Each menu item within the Bottom Navigation View now consists of both an icon and a text label. When a destination is selected, both the icon and label will change to a different color compared to their inactive state.
In Material Design 3, inactive destinations are represented by an outlined version of the icon when applicable. Active destinations are denoted by a filled icon contained within a pill-shaped container.
In cases where no distinct filled and outlined versions of an icon are available, it's advisable to explore alternative methods to signify the active state, such as displaying destination labels exclusively on active destinations.
Floating Action Button
The FloatingActionButton (FAB) has undergone a redesign. In the absence of an explicit theme or when using a legacy Widget.Design.FloatingActionButton theme, the new design is automatically applied within a Theme.Material3.* theme.
The updated FAB features a reduced corner radius and now adopts a rounded rectangle shape, departing from the circular form of previous FABs. Predefined styles are available to theme your FAB using color tones from the Primary, Secondary, or surface colors. To preserve the previous design for your Floating Action Buttons, be sure to set your FAB's style as
@style/Widget.MaterialComponents.FloatingActionButton.
Additionally, a new large FAB variant has been introduced.
Buttons
In Material Design 3, both Filled and Outlined Buttons feature completely rounded corners. Button widgets will automatically adopt a Material Button and apply a Material3 style when placed within a theme derived from Theme.Material3.*. For further guidance on implementing Buttons, including additional examples and code snippets, refer to the documentation.
Chips
In Material Design 2, Chips featured fully rounded corners, but in Material Design 3, they have received a visual update, now sporting 8 dp rounded corners. All Chips now adhere to this 8 dp corner radius in Material Design 3. For comprehensive guidance on implementing Chips, including additional examples and code snippets, consult the documentation.
Badges
The Bottom Navigation View now allows the display of associated badges, either as a filled shape or with a label. You can anchor a badge to one of the four corners of the icon by configuring its gravity using the constants declared in BadgeDrawable.BadgeGravity:
TOP_START TOP_END BOTTOM_START BOTTOM_END
Below is a code example illustrating how to add badges to BottomNavigationView menu items:
java Copy code BottomNavigationView bn = //... reference to your items. // Retrieve the first menu item and increment the badge label MenuItem menuItem = bn.getMenu().getItem(0); int menuItemId = menuItem.getItemId(); BadgeDrawable badgeDrawable = bn.getOrCreateBadge(menuItemId); // If the first menu item is selected and the badge was previously hidden, // use BadgeDrawable.setVisible(true) to ensure the badge becomes visible. badgeDrawable.setVisible(true); badgeDrawable.setNumber(badgeDrawable.getNumber() + 1);
This code snippet demonstrates how to incorporate badges into your BottomNavigationView's menu items.
Conclusion
We strongly recommend upgrading your application's dependencies to fully grasp and explore the fresh possibilities that Material Design 3 offers, allowing you to elevate your user experience through enhanced color capabilities. If you are interested in creating an Android app, don’t hesitate to сontact us, we will be happy to help implement your idea.