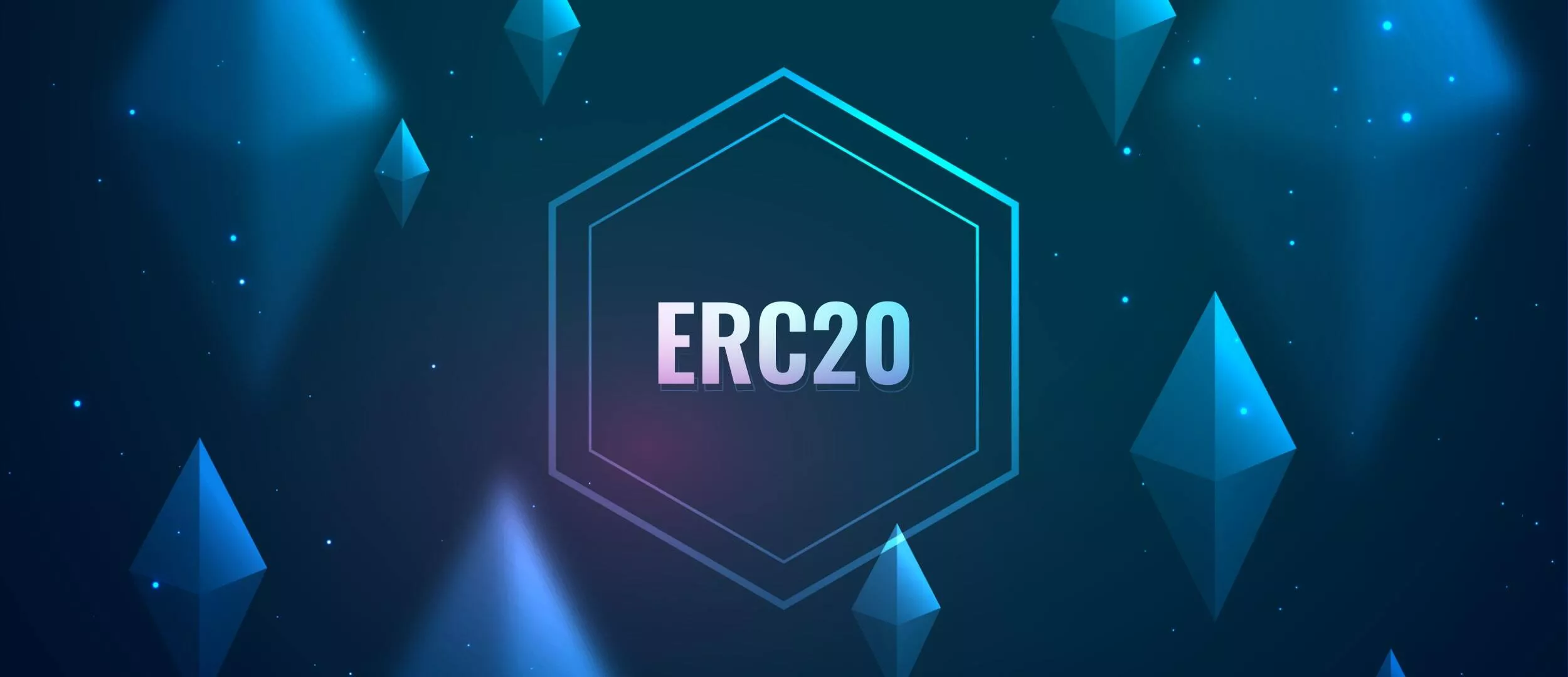
Ethereum is a blockchain platform that allows the creation of decentralized applications (dApps). Both Bitcoin and Ethereum are blockchains. However, both of these blockchains are very different in terms of technical characteristics. Bitcoin offers a peer-to-peer electronic payment system. Ethereum is focused on the execution of program codes of any decentralized application. The popular Ethereum blockchain helps deploy tokens that can be bought, sold, or exchanged. Ethereum was launched in 2015 and has since become the driving force behind the popularity of cryptocurrencies.
In the Ethereum system, tokens represent digital rights. In fact, cryptocurrency units on Ethereum are smart contracts that automatically perform the functions specified in the code and work thanks to the blockchain system. Each coin performs a specific task assigned to it. No one has the right to interfere with the operation of these protocols. When the ERC-20 standard was implemented, Ethereum provided a more organized ecosystem so tokens could operate flawlessly and interact well with dApps and exchanges.
What is an ERC-20 Token?
When it comes to creating tokens, most entrepreneurs choose to issue ERC-20 cryptocurrency units. The Ethereum blockchain provides high flexibility and security. Therefore, it is convenient to develop cryptographic tokens on Ethereum. They are widely used because they can be replaced by other similar tokens.
Ethereum Request for Comments is a set of documents that developers use to deploy protocols. The materials define the rules needed to create tokens in the Ethereum ecosystem. Technical documents are usually written by developers and include information about protocol specifications and contract descriptions. Before becoming a standard, a certain kind of Ethereum Request for Comments must be reviewed, commented on, and accepted by the community through the EIP (Ethereum Improvement Proposal).
The ERC-20 standard resolved the issue by providing a structured framework, enabling wallets and exchanges to easily support and interact with a variety of tokens. This standardization streamlined the integration of tokens, making their exchange and management significantly more seamless.
ERC-20 tokens are cryptocurrencies that perform specified functions. These digital units can have value and process transactions and payments. The Ethereum community created the ERC-20 standard with 5 mandatory and 4 necessary rules.
MANDATORY
Token Name - the name of the token
totalSupply - the total number of ERC-20 units created Symbol - a symbol for exchangers
Transfer - allows you to transfer the number of tokens from the source to the user account
Decimal - up to 18 - (the smallest possible division of the ether is 18 decimal)
NECESSARILY
Approve - checks the transaction against the total number of cryptocurrency units
balanceOf - a function that returns the number of tokens that have the given address in their account
Allowance - checks the user's balance and cancels the operation if there are not enough funds on it
transferFrom - helps to transfer cryptocurrency units to another account
ERC-20 helps streamline the development of Ethereum-based tokens while maintaining their versatility. Thanks to the established standard, new digital assets can be sent to an exchange or transferred to a wallet automatically after deployment.
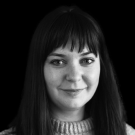
Ivanna
Client Manager
Contact us, and we will share our case studies related to fintech development
Get a Free ConsultationWhy are ERC-20 Tokens So Popular?
Ethereum tokens have gained significant popularity and success due to several key factors:
- ERC-20 tokens are relatively simple to create and deploy. The ERC-20 standard provided the token development process, making it accessible to developers. This simplicity has contributed to the widespread adoption and proliferation of ERC-20 tokens.
- Standardization and Interoperability: The ERC-20 standard addresses a crucial problem in the blockchain ecosystem. It provides a common set of commands that allow blockchain-based marketplaces, exchanges, and wallets to interact with a variety of tokens consistently. This standardization enables seamless integration and interoperability between different tokens, ensuring compatibility and ease of use for users and developers alike.
- Industry Acceptance: While ERC-20 was not the first token specification on Ethereum, its widespread acceptance and adoption within the developer community made it the de facto industry standard. This popularity led to the development of numerous Ethereum tokens, creating a thriving ecosystem of projects, exchanges, and decentralized applications (dApps) built around ERC-20 tokens.
- Token Purchase and Interaction Rules: The ERC-20 standard provides guidelines for token purchase and interaction rules, ensuring consistency and predictability across different tokens. This standardized approach simplifies token transactions, transfers, and token-based operations, making it easier for users to understand and interact with ERC-20 tokens.
How to Create Your Token
Now that we understand ERC-20 tokens and their functionality, let's explore the process of building and deploying our token.
Here we will describe the deployment of the token contract on the Ropsten testnet. You should have the Metamask browser extension for developing an Ethereum (ETH) wallet and obtaining some test ETH.
- Install the Metamask browser extension and create an Ethereum wallet.
- Access the Ropsten Test Network within your Metamask wallet settings.
- Copy your wallet address from Metamask.
- Visit the Ropsten faucet website and locate the text field provided.
- Paste your wallet address into the text field on the faucet webpage.
- Click on the "Send me test Ether" or similar button to receive test ETH in your wallet
Proceed to the Ethereum Remix IDE and create a fresh Solidity file, such as "token.sol.
Here's a simple ERC-20 token contract written in Solidity
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; contract SimpleERC20Token { string publicconstant name ="SimpleToken"; string publicconstant symbol ="ST"; uint8 publicconstant decimals =18; uint256 public totalSupply; mapping(address => uint256)public balanceOf; mapping(address => mapping(address => uint256))public allowance; event Transfer(address indexed from, address indexed to, uint256 value); event Approval(address indexed owner, address indexed spender, uint256 value); constructor(uint256 initialSupply){ totalSupply = initialSupply *(10**uint256(decimals)); balanceOf[msg.sender]= totalSupply;} function transfer(address to, uint256 value) external returns (bool){require(to != address(0),"Invalid recipient address");require(balanceOf[msg.sender]>= value,"Insufficient balance"); balanceOf[msg.sender]-= value; balanceOf[to]+= value; emit Transfer(msg.sender, to, value);returntrue;} function approve(address spender, uint256 value) external returns (bool){require(spender != address(0),"Invalid spender address"); allowance[msg.sender][spender]= value; emit Approval(msg.sender, spender, value);returntrue;} function transferFrom(address from, address to, uint256 value) external returns (bool){require(from != address(0),"Invalid sender address");require(to != address(0),"Invalid recipient address");require(balanceOf[from]>= value,"Insufficient balance");require(allowance[from][msg.sender]>= value,"Allowance exceeded"); balanceOf[from]-= value; balanceOf[to]+= value; allowance[from][msg.sender]-= value; emit Transfer(from, to, value);returntrue;}}
This contract implements a basic ERC-20 token with the following functionalities:
- The name, symbol, and decimals variables are used to define the token's name, symbol, and number of decimal places, respectively.
- The totalSupply variable holds the total supply of the tokens in the contract.
- The balanceOf mapping tracks the token balances of each address.
- The allowance mapping allows token owners to grant approval to other addresses to spend tokens on their behalf.
- The Transfer event is emitted whenever tokens are transferred between addresses.
- The Approval event is emitted whenever approval for token spending is granted to another address.
Please note that this is a basic example for educational purposes and might not include more advanced features or security measures that are typically seen in production-level token contracts. This is the simplest and dangerous contract. real contracts are much larger and more complex, but this one will work too. Always conduct a thorough audit and testing before deploying any smart contract to a live network.
Common Mistakes
If the creator of the cryptocurrency is not a developer or doesn’t know the Solidity language, with a high degree of probability he or she can write the smart contract code incorrectly. The most common mistakes when developing cryptocurrencies on Ethereum are:
throw is deprecated.
This means that there are special functions in the smart contract that should only be called by a specific address designated as the owner. Prior to Solidity 0.4.10 (and sometimes after), this was a common pattern for permission enforcement.
If the useSuperPowers() function is called by anyone other than the owner, the program will throw an error returning an invalid opcode.
In this situation, throw will consume all the gas, while revert will return the unused commission.
Conclusion
Now you have an understanding of how to create an ERC-20 token. Stfalcon specializes in blockchain and token development. We can help you to create an Ethereum token that involves our technical skills. We can explain to you the possibilities and technical aspects of creating an ERC-20 token. Stfalcon developers can build the smart contract code for your ERC-20 token. Our experienced team in Solidity, that utilized for Ethereum smart contracts, can provide adherence to the ERC-20 standard.
We can help to integrate ERC-20 tokens into already existing wallets, DeFi exchanges, and blockchain platforms. Stfalcon can deploy tokens on the Ethereum network on Ropsten. If you are interested in ensuring the successful implementation of your token project, contact us, a free consultation is available.