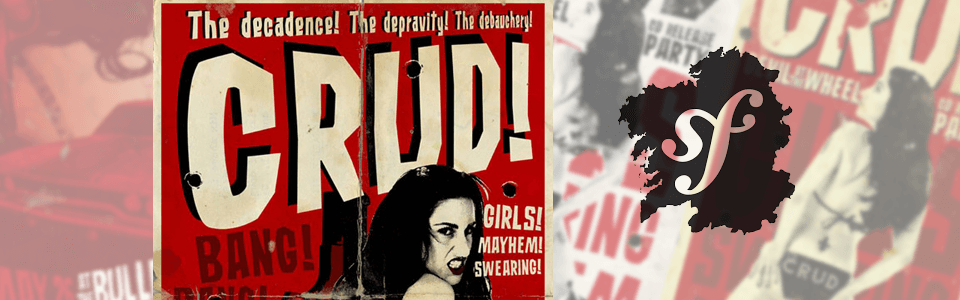
Developing classic CRUD application (Creating/Reading/Updating/Deleting) working with data bases is a pretty typical task. Since a new version of our favorite framework is to be released soon, I’d like to tell you how to create application on Symfony2.
First of all, I’d like to say that Symfony2 fully integrates with Doctrine2 ORM open library that is used for working with data bases. It means that the order for creating CRUD application would be the following: we create bundle, in this bundle we create Doctrine2 ORM entity and then convert it into CRUD application.
Before starting you should check database connection data in configuration. It is usually contained in app/config/parameters.yml. This file is referenced by Doctrine2 ORM main configuration file. It’s convenient to keep all data base connection data in parameters.yml since in this case you can place different versions of this file on different servers.
After you have entered connection data you can tell Doctrine2 ORM that you want to create a data base:
php app/console doctrine:database:create
Creating New Bundle
Symfony2 has a very handy set of console commands for generating various elements. Using them will save you a great deal of time. Since in Symfony2 all features are organized into bundles, you should start by creating a new bundle for your CRUD application:
php app/console generate:bundle
Meta Data
Doctrine2 ORM allows you to persists entire objects into the data base as well as get them out of it. It becomes possible by mapping a PHP class to the data base table and mapping properties of this class to the columns of the data base table:
In order for it to work, you should create meta data that will tell Doctrine2 how to map a class and its properties to a database. This meta data can be provided in different formats (for example, YAML or XML) or directly inside the class using annotations (but you aren’t allowed to provide it in several different formats at once). For example, here’s how you can add meta data for describing Product entity:
// src/Acme/StoreBundle/Entity/Product.php namespace Acme\StoreBundle\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="product") */class Product {/** * @ORM\Id * @ORM\Column(type="integer") * @ORM\GeneratedValue(strategy="AUTO") * * @var int * / private $id;
Creating Entity
The next step in creating CRUD application is to generate entity classes for data manipulation.
php app/console generate:doctrine:entity
Entity is a base class containing data. Please note that it is only possible to generate entities with simple fields so to create connections between entities we need to write down meta data manually in the proper entity classes.
Creating CRUD
Now you can generate a base controller for the entity from the bundle you’ve created. It will allow you to perform 5 operations:
- Get list of all the records.
- Show one record that is defined using its primary key.
- Create a new record.
- Edit an existing record.
- Delete and existing record.
Execute the following command to create the abovementioned controller:
php app/console doctrine:generate:crud
This command has several parameters. It is usually executed in the interactive mode and asks you to provide all the necessary data but if this option is turned off, you have to manually enter values for the following parameters:
--entity
— entity name together with the name of the bundle (BundleName:EntityName).
--route-prefix
— prefix for each routing that defines action.
--with-write
— accepted values: yes|no
. Defines whether to generate new, create, edit, update and delete actions.
--format
— accepted values: annotation|php|yml|xml
. Defines format of the configuration files that will be generated (for example, for routing). If you use annotation format (used by default) make sure you have SensioFrameworkExtraBundle.
--overwrite
— accepted values: yes|no
. Defines whether to overwrite files that already exist. Default value is no
.
That’s all what it takes to create CRUD application on Symfony2. As you can see, it’s quite an easy task.
For a more correct and accurate performance of all elements of the CRUD application we need to spend some time introducing corrections into the form pattern class and adjusting patterns for various pages and forms. But in general creating CRUD application won’t take much of your time.